mirror of
https://github.com/goharbor/harbor
synced 2025-04-08 19:51:33 +00:00
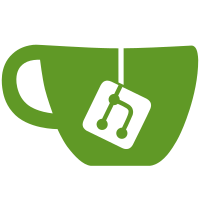
This commit fixes #5040, the harbor-db image will only contain empty databases, and harbor ui container will use migrate tool to run initial SQL scripts to do initialization. This is helpful for the case to configure Harbor against external DB or DBaaS like RDS for HA deployment However, this change will results some confusion as there are two tables to track schema versions have been using alembic for migration, for this release we'll try to use alembic to mock a `migration` table during upgrade so the migrator will be bypassed, in future we'll consider to consolidate to the golang based migrator. Another issue is that the UI and adminserver containers will access DB after start up in different congurations, can't ensure the sequence, so both of them will try to update the schema when started up.
136 lines
3.3 KiB
Go
136 lines
3.3 KiB
Go
// copyright (c) 2017 vmware, inc. all rights reserved.
|
|
//
|
|
// licensed under the apache license, version 2.0 (the "license");
|
|
// you may not use this file except in compliance with the license.
|
|
// you may obtain a copy of the license at
|
|
//
|
|
// http://www.apache.org/licenses/license-2.0
|
|
//
|
|
// unless required by applicable law or agreed to in writing, software
|
|
// distributed under the license is distributed on an "as is" basis,
|
|
// without warranties or conditions of any kind, either express or implied.
|
|
// see the license for the specific language governing permissions and
|
|
// limitations under the license.
|
|
|
|
package dao
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"strconv"
|
|
|
|
"github.com/vmware/harbor/src/common/models"
|
|
"github.com/vmware/harbor/src/common/utils/log"
|
|
)
|
|
|
|
var defaultRegistered = false
|
|
|
|
// PrepareTestForMySQL is for test only.
|
|
func PrepareTestForMySQL() {
|
|
}
|
|
|
|
// PrepareTestForSQLite is for test only.
|
|
func PrepareTestForSQLite() {
|
|
}
|
|
|
|
// PrepareTestForPostgresSQL is for test only.
|
|
func PrepareTestForPostgresSQL() {
|
|
dbHost := os.Getenv("POSTGRESQL_HOST")
|
|
if len(dbHost) == 0 {
|
|
log.Fatalf("environment variable POSTGRESQL_HOST is not set")
|
|
}
|
|
dbUser := os.Getenv("POSTGRESQL_USR")
|
|
if len(dbUser) == 0 {
|
|
log.Fatalf("environment variable POSTGRESQL_USR is not set")
|
|
}
|
|
dbPortStr := os.Getenv("POSTGRESQL_PORT")
|
|
if len(dbPortStr) == 0 {
|
|
log.Fatalf("environment variable POSTGRESQL_PORT is not set")
|
|
}
|
|
dbPort, err := strconv.Atoi(dbPortStr)
|
|
if err != nil {
|
|
log.Fatalf("invalid POSTGRESQL_PORT: %v", err)
|
|
}
|
|
|
|
dbPassword := os.Getenv("POSTGRESQL_PWD")
|
|
dbDatabase := os.Getenv("POSTGRESQL_DATABASE")
|
|
if len(dbDatabase) == 0 {
|
|
log.Fatalf("environment variable POSTGRESQL_DATABASE is not set")
|
|
}
|
|
|
|
database := &models.Database{
|
|
Type: "postgresql",
|
|
PostGreSQL: &models.PostGreSQL{
|
|
Host: dbHost,
|
|
Port: dbPort,
|
|
Username: dbUser,
|
|
Password: dbPassword,
|
|
Database: dbDatabase,
|
|
},
|
|
}
|
|
|
|
log.Infof("POSTGRES_HOST: %s, POSTGRES_USR: %s, POSTGRES_PORT: %d, POSTGRES_PWD: %s\n", dbHost, dbUser, dbPort, dbPassword)
|
|
initDatabaseForTest(database)
|
|
}
|
|
|
|
func initDatabaseForTest(db *models.Database) {
|
|
database, err := getDatabase(db)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
log.Infof("initializing database: %s", database.String())
|
|
|
|
alias := database.Name()
|
|
if !defaultRegistered {
|
|
defaultRegistered = true
|
|
alias = "default"
|
|
}
|
|
if err := database.Register(alias); err != nil {
|
|
panic(err)
|
|
}
|
|
if err := database.UpgradeSchema(); err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
if alias != "default" {
|
|
if err = globalOrm.Using(alias); err != nil {
|
|
log.Fatalf("failed to create new orm: %v", err)
|
|
}
|
|
}
|
|
}
|
|
|
|
// PrepareTestData -- Clean and Create data
|
|
func PrepareTestData(clearSqls []string, initSqls []string) {
|
|
o := GetOrmer()
|
|
|
|
for _, sql := range clearSqls {
|
|
fmt.Printf("Exec sql:%v\n", sql)
|
|
_, err := o.Raw(sql).Exec()
|
|
if err != nil {
|
|
fmt.Printf("failed to clear database, sql:%v, error: %v", sql, err)
|
|
}
|
|
}
|
|
|
|
for _, sql := range initSqls {
|
|
_, err := o.Raw(sql).Exec()
|
|
if err != nil {
|
|
fmt.Printf("failed to init database, sql:%v, error: %v", sql, err)
|
|
}
|
|
}
|
|
}
|
|
|
|
// ArrayEqual ...
|
|
func ArrayEqual(arrayA, arrayB []int) bool {
|
|
if len(arrayA) != len(arrayB) {
|
|
return false
|
|
}
|
|
size := len(arrayA)
|
|
for i := 0; i < size; i++ {
|
|
if arrayA[i] != arrayB[i] {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|