mirror of
https://github.com/ohmyzsh/ohmyzsh.git
synced 2024-09-20 15:55:32 +00:00
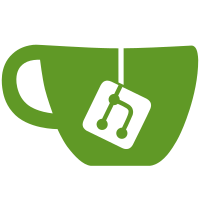
Before, when typing the `marks` command, longer mark keys would cause the tabs to spill over to the next tab stop, like so: rc -> /home/ahlex/.rc repos -> /home/ahlex/repos a-longer-string -> /tmp Implement better key display by running through all of the marks twice, once to get the longest key length, and the second time to format everything according to that length: rc -> /home/ahlex/.rc repos -> /home/ahlex/repos a-longer-string -> /tmp
65 lines
1.5 KiB
Bash
65 lines
1.5 KiB
Bash
# Easily jump around the file system by manually adding marks
|
|
# marks are stored as symbolic links in the directory $MARKPATH (default $HOME/.marks)
|
|
#
|
|
# jump FOO: jump to a mark named FOO
|
|
# mark FOO: create a mark named FOO
|
|
# unmark FOO: delete a mark
|
|
# marks: lists all marks
|
|
#
|
|
export MARKPATH=$HOME/.marks
|
|
|
|
jump() {
|
|
cd -P "$MARKPATH/$1" 2>/dev/null || {echo "No such mark: $1"; return 1}
|
|
}
|
|
|
|
mark() {
|
|
if [[ ( $# == 0 ) || ( "$1" == "." ) ]]; then
|
|
MARK=$(basename "$PWD")
|
|
else
|
|
MARK="$1"
|
|
fi
|
|
if read -q \?"Mark $PWD as ${MARK}? (y/n) "; then
|
|
mkdir -p "$MARKPATH"; ln -sfn "$PWD" "$MARKPATH/$MARK"
|
|
fi
|
|
}
|
|
|
|
unmark() {
|
|
rm -i "$MARKPATH/$1"
|
|
}
|
|
|
|
marks() {
|
|
local max=0
|
|
for link in $MARKPATH/*(@); do
|
|
if [[ ${#link:t} -gt $max ]]; then
|
|
max=${#link:t}
|
|
fi
|
|
done
|
|
local printf_markname_template="$(printf -- "%%%us " "$max")"
|
|
for link in $MARKPATH/*(@); do
|
|
local markname="$fg[cyan]${link:t}$reset_color"
|
|
local markpath="$fg[blue]$(readlink $link)$reset_color"
|
|
printf -- "$printf_markname_template" "$markname"
|
|
printf -- "-> %s\n" "$markpath"
|
|
done
|
|
}
|
|
|
|
_completemarks() {
|
|
if [[ $(ls "${MARKPATH}" | wc -l) -gt 1 ]]; then
|
|
reply=($(ls $MARKPATH/**/*(-) | grep : | sed -E 's/(.*)\/([_a-zA-Z0-9\.\-]*):$/\2/g'))
|
|
else
|
|
if readlink -e "${MARKPATH}"/* &>/dev/null; then
|
|
reply=($(ls "${MARKPATH}"))
|
|
fi
|
|
fi
|
|
}
|
|
compctl -K _completemarks jump
|
|
compctl -K _completemarks unmark
|
|
|
|
_mark_expansion() {
|
|
setopt extendedglob
|
|
autoload -U modify-current-argument
|
|
modify-current-argument '$(readlink "$MARKPATH/$ARG")'
|
|
}
|
|
zle -N _mark_expansion
|
|
bindkey "^g" _mark_expansion
|