mirror of
https://github.com/wesnoth/wesnoth
synced 2025-04-20 20:16:46 +00:00
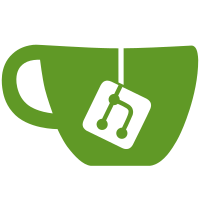
It's mostly about making the scripts run if python defaults to python3. Has been tested for each script.
53 lines
1.3 KiB
Python
Executable File
53 lines
1.3 KiB
Python
Executable File
#!/usr/bin/env python2
|
|
|
|
"""
|
|
This script is used to detect functions in the source code which are no longer
|
|
used. It should be used after compiling all the different executables with the
|
|
following flags:
|
|
|
|
CXXFLAGS += "-ffunction-sections"
|
|
LDFLAGS += "-Wl,-gc-sections"
|
|
|
|
It will then use nm to get all "T" symbols from all .o files generated by
|
|
compilation, and compare those symbols to the symbols in the executables.
|
|
Symbols not in any executable likely are unused.
|
|
"""
|
|
|
|
import os, glob
|
|
|
|
def nm(filename):
|
|
return os.popen("nm -C %s" % filename).read()
|
|
|
|
output1 = []
|
|
for o in glob.glob("src/*.o") + glob.glob("src/*/*.o") + \
|
|
glob.glob("src/*/*/*.o") + glob.glob("src/*/*/*/*.o"):
|
|
output1.append((o, nm(o)))
|
|
|
|
output2 = nm("src/wesnoth")
|
|
output2 += nm("src/campaignd")
|
|
output2 += nm("src/exploder")
|
|
output2 += nm("src/cutter")
|
|
output2 += nm("src/wesnothd")
|
|
output2 += nm("src/test")
|
|
|
|
def extract(line):
|
|
return line[line.find(" T ") + 3:]
|
|
|
|
def symbols(lines):
|
|
return [extract(x) for x in lines.splitlines() if " T " in x]
|
|
|
|
symbols2 = symbols(output2)
|
|
|
|
for o in output1:
|
|
symbols1 = symbols(o[1])
|
|
|
|
found = []
|
|
for symbol in symbols1:
|
|
if not symbol in symbols2:
|
|
found += [symbol]
|
|
|
|
if found:
|
|
print "%s:" % o[0]
|
|
print "\n".join(found)
|
|
print
|