mirror of
https://github.com/wesnoth/wesnoth
synced 2025-04-24 04:40:45 +00:00
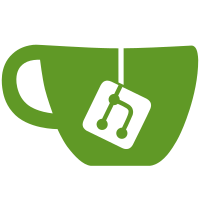
It's mostly about making the scripts run if python defaults to python3. Has been tested for each script.
44 lines
1.2 KiB
Python
Executable File
44 lines
1.2 KiB
Python
Executable File
#!/usr/bin/env python2
|
|
# codelist
|
|
# given list of integers, one per line, outputs a minimal list of ranges
|
|
# describing the list
|
|
|
|
# this is useful for codepoints that are in a font, based on list of codes
|
|
# output format is suitable for codepoints="" in Wesnoth fonts.cfg
|
|
|
|
import sys
|
|
|
|
|
|
def rangeify(lst):
|
|
"""
|
|
Turn ranges of adjacent ints in a list into [start, end] list elements.
|
|
"""
|
|
lst.sort()
|
|
lst.append(None)
|
|
while True:
|
|
for i in range(len(lst) - 1):
|
|
if isinstance(lst[i], int) and lst[i + 1] == lst[i] + 1:
|
|
lst = lst[:i] + [[lst[i], lst[i + 1]]] + lst[i + 2:]
|
|
break
|
|
elif isinstance(lst[i], list) and lst[i + 1] == lst[i][-1] + 1:
|
|
lst[i][1] = lst[i + 1]
|
|
lst = lst[:i + 1] + lst[i + 2:]
|
|
break
|
|
else:
|
|
break
|
|
lst.pop()
|
|
return lst
|
|
|
|
|
|
def printbyrange(lst):
|
|
out = ""
|
|
for elt in lst:
|
|
if isinstance(elt, int):
|
|
out += repr(elt) + ","
|
|
else:
|
|
out += "%d-%d," % tuple(elt)
|
|
return out[:-1]
|
|
|
|
codepoints = [int(x.strip) for x in sys.stdin.readlines()]
|
|
print printbyrange(rangeify(codepoints))
|