mirror of
https://github.com/wesnoth/wesnoth
synced 2025-05-17 22:03:24 +00:00
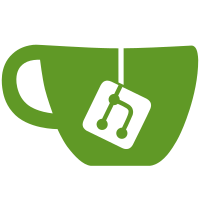
This looks safe to me, and now that we're early in 1.5 we'll have time to notice and fix any problems it creates.
51 lines
1.4 KiB
Python
51 lines
1.4 KiB
Python
import re, os, safe
|
|
|
|
whitelisted = ["wesnoth", "heapq", "random", "math", "string", "re", "threading"]
|
|
rex = re.compile(r"^import\s+(.*)", re.M)
|
|
modules = {}
|
|
|
|
def include(matchob):
|
|
"""
|
|
Regular expression callback. Handles a single import statement, returning
|
|
the included code.
|
|
"""
|
|
names = [x.strip() for x in matchob.group(1).split(",")]
|
|
r = ""
|
|
for name in names:
|
|
if name in whitelisted:
|
|
modules[name] = __import__(name)
|
|
continue
|
|
for path in pathes:
|
|
includefile = os.path.join(path, name)
|
|
try:
|
|
code = parse_file(includefile + ".py")
|
|
break
|
|
except IOError:
|
|
pass
|
|
else:
|
|
raise safe.SafeException("Could not include %s." % name)
|
|
return None
|
|
r += code
|
|
return r
|
|
|
|
def parse_file(name):
|
|
"""
|
|
Simple pre-parsing of scripts, all it does is allow importing other scripts.
|
|
"""
|
|
abspath = os.path.abspath(name)
|
|
if abspath in already: return ""
|
|
already[abspath] = 1
|
|
code = file(abspath).read().replace(chr(13), "")
|
|
code = rex.sub(include, code)
|
|
return code
|
|
|
|
# If you want to disable safe python, use this instead:
|
|
#
|
|
# def parse(name): return open(name).read(), {}
|
|
def parse(name):
|
|
global already, modules
|
|
already = {}
|
|
modules = {}
|
|
return parse_file(name), modules
|
|
|