mirror of
https://github.com/wesnoth/wesnoth
synced 2025-05-07 04:36:41 +00:00
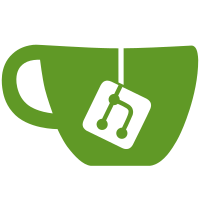
This (should) allow sdl/utils.hpp to be modified without a huge rebuild, since a number of widely used headers included that file simply for the surface class type.
149 lines
2.9 KiB
C++
149 lines
2.9 KiB
C++
/*
|
|
Copyright (C) 2014 - 2016 by Mark de Wever <koraq@xs4all.nl>
|
|
Part of the Battle for Wesnoth Project http://www.wesnoth.org/
|
|
|
|
This program is free software; you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation; either version 2 of the License, or
|
|
(at your option) any later version.
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY.
|
|
|
|
See the COPYING file for more details.
|
|
*/
|
|
|
|
#include "sdl/surface.hpp"
|
|
#include "sdl/window.hpp"
|
|
|
|
#include "sdl/exception.hpp"
|
|
|
|
#include <SDL_render.h>
|
|
|
|
namespace sdl
|
|
{
|
|
|
|
window::window(const std::string& title,
|
|
const int x,
|
|
const int y,
|
|
const int w,
|
|
const int h,
|
|
const Uint32 window_flags,
|
|
const Uint32 render_flags)
|
|
: window_(SDL_CreateWindow(title.c_str(), x, y, w, h, window_flags))
|
|
, pixel_format_(SDL_PIXELFORMAT_UNKNOWN)
|
|
{
|
|
if(!window_) {
|
|
throw exception("Failed to create a SDL_Window object.", true);
|
|
}
|
|
|
|
if(!SDL_CreateRenderer(window_, -1, render_flags)) {
|
|
throw exception("Failed to create a SDL_Renderer object.", true);
|
|
}
|
|
|
|
SDL_RendererInfo info;
|
|
if(SDL_GetRendererInfo(*this, &info) != 0) {
|
|
throw exception("Failed to retrieve the information of the renderer.",
|
|
true);
|
|
}
|
|
|
|
if(info.num_texture_formats == 0) {
|
|
throw exception("The renderer has no texture information available.\n",
|
|
false);
|
|
}
|
|
|
|
pixel_format_ = info.texture_formats[0];
|
|
|
|
fill(0,0,0);
|
|
|
|
render();
|
|
}
|
|
|
|
window::~window()
|
|
{
|
|
if(window_) {
|
|
SDL_DestroyWindow(window_);
|
|
}
|
|
}
|
|
|
|
void window::set_size(const int w, const int h)
|
|
{
|
|
SDL_SetWindowSize(window_, w, h);
|
|
}
|
|
|
|
void window::center()
|
|
{
|
|
SDL_SetWindowPosition(window_, SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED);
|
|
}
|
|
|
|
void window::maximize()
|
|
{
|
|
SDL_MaximizeWindow(window_);
|
|
}
|
|
|
|
void window::to_window()
|
|
{
|
|
SDL_SetWindowFullscreen(window_, 0);
|
|
}
|
|
|
|
void window::restore()
|
|
{
|
|
SDL_RestoreWindow(window_);
|
|
}
|
|
|
|
void window::full_screen()
|
|
{
|
|
SDL_SetWindowFullscreen(window_, SDL_WINDOW_FULLSCREEN_DESKTOP);
|
|
}
|
|
|
|
void window::fill(Uint8 r, Uint8 g, Uint8 b, Uint8 a)
|
|
{
|
|
SDL_SetRenderDrawColor(*this, r, g, b, a);
|
|
if(SDL_RenderClear(*this) != 0) {
|
|
throw exception("Failed to clear the SDL_Renderer object.",
|
|
true);
|
|
}
|
|
}
|
|
|
|
void window::render()
|
|
{
|
|
SDL_RenderPresent(*this);
|
|
}
|
|
|
|
void window::set_title(const std::string& title)
|
|
{
|
|
SDL_SetWindowTitle(window_, title.c_str());
|
|
}
|
|
|
|
void window::set_icon(const surface& icon)
|
|
{
|
|
SDL_SetWindowIcon(window_, icon);
|
|
}
|
|
|
|
int window::get_flags()
|
|
{
|
|
return SDL_GetWindowFlags(window_);
|
|
}
|
|
|
|
void window::set_minimum_size(int min_w, int min_h)
|
|
{
|
|
SDL_SetWindowMinimumSize(window_, min_w, min_h);
|
|
}
|
|
|
|
int window::get_display_index()
|
|
{
|
|
return SDL_GetWindowDisplayIndex(window_);
|
|
}
|
|
|
|
window::operator SDL_Window*()
|
|
{
|
|
return window_;
|
|
}
|
|
|
|
window::operator SDL_Renderer*()
|
|
{
|
|
return SDL_GetRenderer(window_);
|
|
}
|
|
|
|
} // namespace sdl
|
|
|