mirror of
https://github.com/wesnoth/wesnoth
synced 2025-05-11 17:28:18 +00:00
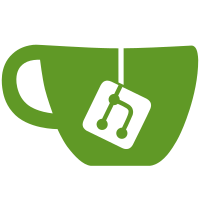
- added new status image for illuminated underground - made Li'sar start at level 2 and be upgradeable to level 3 - made it so users in lobby can see minimap of non-shroud games being played - made it so the 'observer' setting is respected for multiplayer games - made it so that the number of available positions in a game is shown in the lobby - made it so the current turn of a game underway is shown in the lobby - made the 'eye of the observer' work, allowing players to see who is observing their game. (only updates when it's a network player's turn)
84 lines
3.0 KiB
C++
84 lines
3.0 KiB
C++
#ifndef IMAGE_HPP_INCLUDED
|
|
#define IMAGE_HPP_INCLUDED
|
|
|
|
#include "map.hpp"
|
|
#include "unit.hpp"
|
|
|
|
#include "SDL.h"
|
|
#include <string>
|
|
|
|
class team;
|
|
|
|
//this module manages the cache of images. With an image name, you can get
|
|
//the surface corresponding to that image, and don't need to free the image.
|
|
//Note that surfaces returned from here are invalidated whenever events::pump()
|
|
//is called, and so shouldn't be kept, but should be regotten from here as
|
|
//needed.
|
|
//
|
|
//images come in a number of varieties:
|
|
// - unscaled: no modifications have been done on the image.
|
|
// - scaled: images are scaled to the size of a tile
|
|
// - greyed: images are scaled and in greyscale
|
|
// - brightened: images are scaled and brighter than normal.
|
|
namespace image {
|
|
|
|
//the image manager is responsible for setting up images, and destroying
|
|
//all images when the program exits. It should probably
|
|
//be created once for the life of the program
|
|
struct manager
|
|
{
|
|
manager();
|
|
~manager();
|
|
};
|
|
|
|
//function to set the program's icon to the window manager.
|
|
//must be called after SDL_Init() is called, but before setting the
|
|
//video mode
|
|
void set_wm_icon();
|
|
|
|
//will make all scaled images have these rgb values added to all
|
|
//their pixels. i.e. add a certain colour hint to images. useful
|
|
//for representing day/night. Invalidates all scaled images.
|
|
void set_colour_adjustment(int r, int g, int b);
|
|
|
|
//function to get back the current colour adjustment values
|
|
void get_colour_adjustment(int *r, int *g, int *b);
|
|
|
|
//sets the pixel format used by the images. Is called every time the
|
|
//video mode changes. Invalidates all images.
|
|
void set_pixel_format(SDL_PixelFormat* format);
|
|
|
|
//sets the amount scaled images should be scaled. Invalidates all
|
|
//scaled images.
|
|
void set_zoom(double zoom);
|
|
|
|
enum TYPE { UNSCALED, SCALED, GREYED, BRIGHTENED };
|
|
|
|
enum COLOUR_ADJUSTMENT { ADJUST_COLOUR, NO_ADJUST_COLOUR };
|
|
|
|
//function to get the surface corresponding to an image.
|
|
//note that this surface must be freed by the user by calling
|
|
//SDL_FreeSurface
|
|
SDL_Surface* get_image(const std::string& filename,TYPE type=SCALED, COLOUR_ADJUSTMENT adj=ADJUST_COLOUR);
|
|
|
|
//function to get a scaled image, but scale it to specific dimensions.
|
|
//if you later try to get the same image using get_image() the image will
|
|
//have the dimensions specified here.
|
|
//Note that this surface must be freed by the user by calling SDL_FreeSurface
|
|
SDL_Surface* get_image_dim(const std::string& filename, size_t x, size_t y);
|
|
|
|
//function to register an image with the given id. Calls to get_image(id,UNSCALED) will
|
|
//return this image. register_image() will take ownership of this image and free
|
|
//it when the cache is cleared (change of video mode or colour adjustment).
|
|
//If there is already an image registered with this id, that image will be freed
|
|
//and replaced with this image.
|
|
void register_image(const std::string& id, SDL_Surface* surf);
|
|
|
|
//the surface returned must be freed by the user
|
|
SDL_Surface* getMinimap(int w, int h, const gamemap& map_, int lawful_bonus,
|
|
const team* tm=NULL);
|
|
}
|
|
|
|
#endif
|
|
|